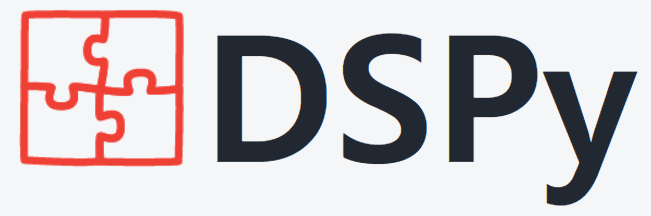
DSPy 모듈은 LM을 사용하는 프로그램을 위한 빌딩 블록입니다.
내장된 각 모듈은 프롬프트 기법(예: 생각의 사슬 또는 ReAct)을 추상화합니다. 결정적으로, 이 모듈은 모든 DSPy Signature.을 처리하도록 일반화되어 있습니다.
DSPy 모듈에는 학습 가능한 매개변수(즉, 프롬프트와 LM 가중치를 구성하는 작은 조각)가 있으며 입력을 처리하고 출력을 반환하기 위해 호출(호출)할 수 있습니다.
여러 모듈을 더 큰 모듈(프로그램)로 구성할 수 있습니다. DSPy 모듈은 PyTorch의 NN 모듈에서 직접 영감을 받았지만 LM 프로그램에 적용됩니다.
dspy.Predict 또는 dspy.ChainOfThought와 같은 기본 제공 모듈은 어떻게 사용하나요?
가장 기본적인 모듈인 dspy.Predict부터 시작해 보겠습니다. 내부적으로 다른 모든 DSPy 모듈은 dspy.Predict를 사용하여 빌드됩니다.
DSPy에서 사용하는 모듈의 동작을 정의하기 위한 선언적 사양인 DSPy signatures에 대해 이미 어느 정도 알고 있다고 가정하겠습니다.
모듈을 사용하려면 먼저 모듈에 서명을 부여하여 모듈을 선언합니다. 그런 다음 입력 인수를 사용하여 모듈을 호출하고 출력 필드를 추출합니다!
# SST-2 dataset. 예제
sentence = "it's a charming and often affecting journey."
# 1) 서명을 정의
classify = dspy.Predict('sentence -> sentiment')
# 2) input argument(s) 호출
response = classify(sentence=sentence)
# 3) Access the output.
print(response.sentiment)
Output:
Positive
모듈을 선언할 때 모듈에 구성 키를 전달할 수 있습니다.
아래에서는 n=5를 전달하여 5개의 완료를 요청합니다. 온도 또는 max_len 등을 전달할 수도 있습니다.
dspy.ChainOfThought를 사용해 봅시다. 많은 경우 dspy.Predict 대신 dspy.ChainOfThought를 교체하는 것만으로도 품질이 향상됩니다.
question question = "What's something great about the ColBERT retrieval model?"
# 1) 서명과 함께 선언하고 몇 가지 설정을 전달합니다.
classify = dspy.ChainOfThought('question -> answer', n=5)
# 2) 입력 인수를 사용하여 호출합니다.
response = classify(question=question)
# 3) Access the outputs.
response.completions.answer
Output:
['One great thing about the ColBERT retrieval model is its superior efficiency and effectiveness compared to other models.',
'Its ability to efficiently retrieve relevant information from large document collections.',
'One great thing about the ColBERT retrieval model is its superior performance compared to other models and its efficient use of pre-trained language models.',
'One great thing about the ColBERT retrieval model is its superior efficiency and accuracy compared to other models.',
'One great thing about the ColBERT retrieval model is its ability to incorporate user feedback and support complex queries.']
여기서 출력 객체에 대해 설명하겠습니다.
dspy.ChainOfThought 모듈은 일반적으로 서명의 출력 필드 앞에 근거를 삽입합니다
(첫 번째) 근거를 살펴보고 답변을 살펴보겠습니다!
print(f"Rationale: {response.rationale}")
print(f"Answer: {response.answer}")
Output:
Rationale: produce the answer. We can consider the fact that ColBERT has shown to outperform other state-of-the-art retrieval models in terms of efficiency and effectiveness. It uses contextualized embeddings and performs document retrieval in a way that is both accurate and scalable.
Answer: One great thing about the ColBERT retrieval model is its superior efficiency and effectiveness compared to other models.
이 기능은 하나 또는 여러 개의 완료를 요청할 때 액세스할 수 있습니다.
또한 다양한 완성도를 예측 목록 또는 각 필드에 대해 하나씩 여러 개의 목록으로 액세스할 수도 있습니다.
response.completions[3].rationale == response.completions.rationale[3]
Output:
True
다른 DSPy 모듈에는 어떤 것이 있나요? 어떻게 사용할 수 있나요?
다른 것들도 매우 유사합니다. 이들은 주로 서명이 구현되는 내부 동작을 변경합니다!
- dspy.Predict: 기본 예측자. 서명을 수정하지 않습니다. 학습의 주요 형태(예: 지침 및 데모 저장, LM에 대한 업데이트)를 처리합니다.
- dspy.ChainOfThought: 서명의 응답을 커밋하기 전에 LM이 단계별로 생각하도록 가르칩니다.
- dspy.ProgramOfThought: 실행 결과가 응답을 지시하는 코드를 출력하도록 LM을 가르칩니다.
- dspy.ReAct: 주어진 서명을 구현하기 위해 도구를 사용할 수 있는 에이전트입니다.
- dspy.MultiChainComparison: ChainOfThought의 여러 출력을 비교하여 최종 예측을 생성할 수 있습니다.
- 기능 스타일 모듈도 몇 가지 있습니다:
- dspy.majority: 기본 투표를 수행하여 일련의 예측에서 가장 인기 있는 응답을 반환할 수 있습니다.
여러 모듈을 더 큰 프로그램으로 구성하려면 어떻게 해야 하나요?
DSPy는 원하는 제어 흐름에서 모듈을 사용하는 파이썬 코드일 뿐입니다. (컴파일 시 내부적으로 LM 호출을 추적하는 마법이 있습니다).
즉, 모듈을 자유롭게 호출할 수 있다는 뜻입니다. 호출 연쇄를 위한 이상한 추상화가 필요 없습니다.
이것은 기본적으로 실행별 정의/동적 계산 그래프에 대한 PyTorch의 설계 방식입니다. 예제는 소개 튜토리얼을 참조하세요.